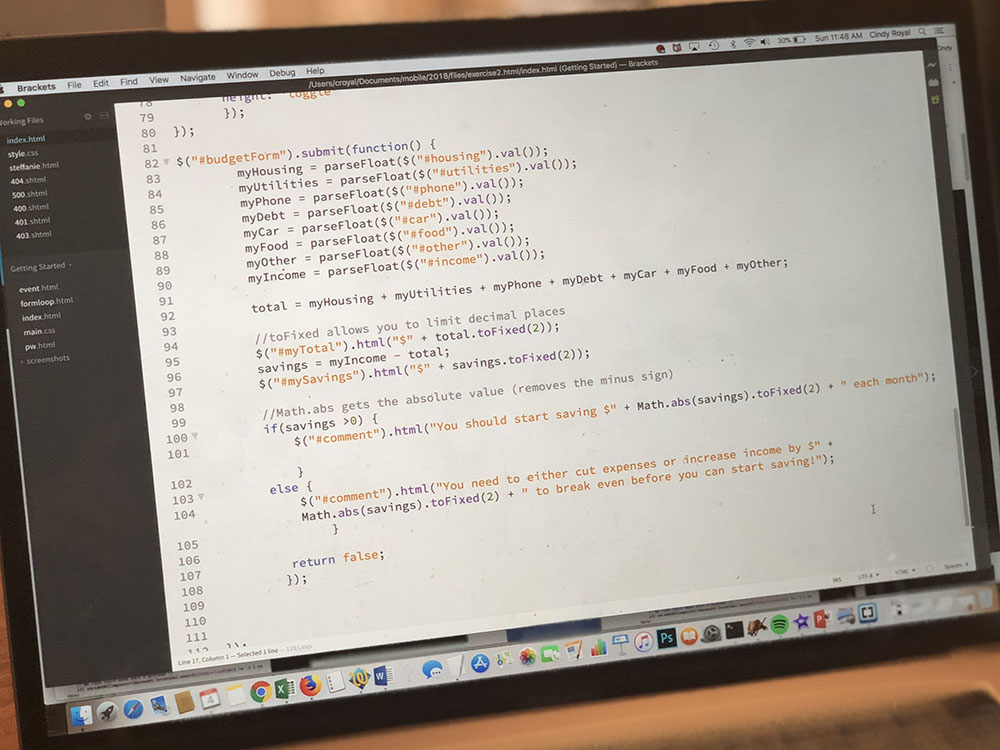
Instructor: Cindy Royal
Friday, July 1, 1-5pm
Description
In this module, you will learn more about programming concepts and apply them in a hands-on exercise.
Outline
I. Overview of HTML/CSS/Bootstrap Concepts
II. Intro to Programming
III. DOM Manipulation
IV. Quiz Exercise
V. How to Set Up a Web Design Class
VI. Google Charts Exercise
VII. Other things you can do if you know some basic code
JavaScript Interactivity Examples
I will be reviewing the Interactive Examples Glitch Project. Feel free to remix this for your own purposes, but I will just be demonstrating in class.
Interactive Quiz Exercise
We will be using a variety of HTML form elements to create a quiz that will provide a score to the user. Find the PhDigital Exercises Project on Glitch and Remix it to your own Glitch account. We will be working with this project as a template. You will have code samples to paste into it to create an interactive quiz. Try to follow along with the exercise. If you get lost, you can go back and review the steps and troubleshoot any issues.
The index.html page is the Quiz page. There is a css page that includes a few styles to control the look of the page that will be applied to both pages we work on today. We will use the chart.html file for the 2nd exercise.
Forms
There are two forms on the index.html (quiz) page. One is a simple form that asks the user’s name. We can use this name in other places in the Quiz to personalize the results.
The first form, form1, has an opening and closing form element, a label and an input of type text. An id of “yourname” has been established to identify the user’s input. I put this in a <p> tag to format it, but you can use any elements or styles to contain these elements. A button with type ‘submit’ is used to Submit the form. Once the value has been captured, it can be used in the page.
DOM Manipulation
The Document Object Model is a representation of the structure and content of the page. To manipulate an element in the DOM, you need a DOM element in which to send the result. Below the form, you will see an empty paragraph with an id of “name”. This will not be visible until the user submits the form. However, if you wanted to start with text in this paragraph, it will be replaced when the form is executed via the script below.
<p id="name"></p>
Script
Let’s create the script for form1, capturing the user’s name and responding. Below the html items, find the script element to include our JavaScript. JavaScript works natively in modern browsers without any additional links or plugins required. Include this code within the script element already provided (don’t repeat the opening and closing script tags).
<script>
document.getElementById("form1").onsubmit=function() {
yrname = document.getElementById("yourname").value;
document.getElementById("name").innerHTML = "Welcome, " + yrname + "!" ;
return false;
}
</script>
The first line provides the code for the event on which the script is executed, in this case the “onsubmit” event. We have created a generic function that gets the value of “yourname”, assigns to a variable named “yrname” and then manipulates the DOM element of “name” with some text concatenated with the “yrname” variable.
The return false; is required to prevent the Submit functions default behavior of refreshing the page. We simply want to manipulate an element on the page. This is not necessary for other types of events.
- Try it and submit the form with your name. If you have problems, use the Chrome Inspector Console to see if it can help you troubleshoot. The Console will often highlight if there is a syntax problem, indicating the row number.
- Change some of the text that is concatenated with the variable in the code and try it again.
Congratulations! You have performed a DOM manipulation.
Show/Hide a Div
Notice that we have a paragraph in a div with an id of “quiz.” By doing so, we can manipulate the DOM to hide the quiz, until the user inputs her name.
<div id="quiz">
<p>How well do you know Covid-19?...</p>
...Rest of quiz
</div>
At the top of the page, find the <style> section. For this element, we want to initially have the quiz hidden with CSS. Include this in the style area.
#quiz {
display: none;
}
Refresh the page again and that the “quiz” div is now hidden.
Let’s add a line of code to the form1 function to have it appear when that code is executed. Put this above the return false; statement.
document.getElementById("quiz").style.display = "block";
This code changes the display style from none to block, so it is now showing. Refresh the page to be sure it is working.
Coding the Quiz
Look at the html. We are giving each answer its own id (q1, q2, q3, q4), to assign the value provided to a variable. Notice that each select grouping has values assigned to each option. The correct answer gets 25 points, incorrect gets 0. Since there are four questions, 25 x 4 = 100!
There are also two DOM elements after the form is closed. They will be used to hold our results.
Scripting the Quiz
In your scripting area, below where we have been previously working, include this code for form2. This is a separate function for form2.
document.getElementById("form2").onsubmit=function() {
total = 0;
q1 = parseInt(document.getElementById("q1").value);
q2 = parseInt(document.getElementById("q2").value);
q3 = parseInt(document.getElementById("q3").value);
q4 = parseInt(document.getElementById("q4").value);
total = q1 + q2 + q3 + q4;
document.getElementById("score").innerHTML = "Your score is " + total;
return false;
}
Let’s look at what is happening in the code. In this area, we are initializing a variable named “total” at 0, so it resets every time the form is submitted. We get the values from each select grouping, but they need to be understood as integers, thus the parseInt statement. A total is calculated. And a score is provided in the total DOM element when the form is submitted.
Check your project in Glitch. If you have problems, use the Chrome Inspector Console (ctrl-click on page and select Inspect from context menu and choose Console) or look for errors in the code on Glitch to see if it can help you troubleshoot.
Adding a Response using JSON
JSON or JavaScript Object Notation is a way to store data in a text format on the web. It is an array of objects, holding key/value pairs. You can use a loop to traverse the JSON to filter the data to a specific request. In this case, we are going to use the score and compare it to a value in the JSON to provide a response to the user, as well as a meme. The images are already available in the img folder in the Bootstrap installation.
Grab the following data and include in the form2 function, above the return false; statement. We are providing links to Giphy memes, but If you were using images, you would use the direct reference to images in your Assets folder.
feedback = [
{total: 0, response: "Zero. You need to learn more about the Covid-19 pandemic.", img: "https://media.giphy.com/media/CfbDPJ17xZwqI/giphy.gif"},
{total: 25, response: "Poor. Needs improvement. Study up so you have good info about the disease.", img: "https://media.giphy.com/media/26gsetuXxUjaWPLA4/giphy.gif"},
{total: 50, response: "Fair. Your understanding of the pandemic could be better.", img: "https://media.giphy.com/media/ggu8E0UeH7bINjvacc/giphy-downsized-large.gif"},
{total: 75, response: "Good. You have a good understanding of Covid-19.", img: "https://media.giphy.com/media/BrFuiMe3YUt3laSeEO/giphy.gif"},
{total: 100, response: "Excellent. You know a lot about the Covid-19 coronavirus pandemic.", img: "https://media.giphy.com/media/l2Je19AZkPF7r6gXm/giphy.gif"},
];
for(i=0;i<feedback.length; i++) {
if(total == feedback[i].total){
document.getElementById("answer").innerHTML = feedback[i].response + "<br><img src=" + feedback[i].img + " >" ;
}
}
Notice the for loop that uses the iterator “i” to traverse each item in the JSON. Using the if statement, when it finds a match with the value of score, it provides the response. And if you have the images, it provides the meme image and establishes a width, concatenating html into the answer.
Try the project again in Glitch. You should be able to take the quiz and see the response and image based on your score. If you have problems, use the Chrome Inspector Console to see if it can help you troubleshoot or see if the Glitch code has any errors.
All of these items can be adjusted for your own purposes and layout.
Exercise:
- Make sure you have a working quiz with the code provided above. If you have problems, use the Chrome Inspector Console or the Glitch code hints to see if it can help you troubleshoot.
- Add a question to this quiz (see the html below. What do you need to change to make it work with 5 questions? Consider the question’s unique id, the point values, the way the value is assigned and added to total, and how the json works to report the result. Create another response and find another giphy link to represent an Average score value (between Good and Fair).
<p>Staying apart from other people when you have been exposed to the coronavirus is called:</p>
<select id="q5">
<option value="0">Choose:</option>
<option value="0">Physical Distancing</option>
<option value="0">Quarantining</option> <!-- this is the correct answer -->
<option value="0">Isolation</option>
</select>
- Can you concatenate the user’s name into the area where you provide the score? Remember, we captured the users name with the first form in the yrname variable.
- What happens if the user forgets to answer a question? How could you handle that? Just think about the options that would make sense in this situation. Discuss with your group and we will review.
Congrats! You have created a functioning interactive quiz that provides responses to a user based on her answers! Our next module will look at making interactive charts. You’ll notice now that the link from the index.html page for the quiz will open this quiz. You are starting to make an interactive product/application.
Next we’ll talk to Sara about creating a Web Design course. Then we’ll pick up with an additional exercise for making an interactive chart.
Google Charts Exercise
The Google Charts API provides code that you can use, with your knowledge of JavaScript, to make interactive charts. Look through the extensive Google Chart Gallery for ideas about charts you’d might be able to use.
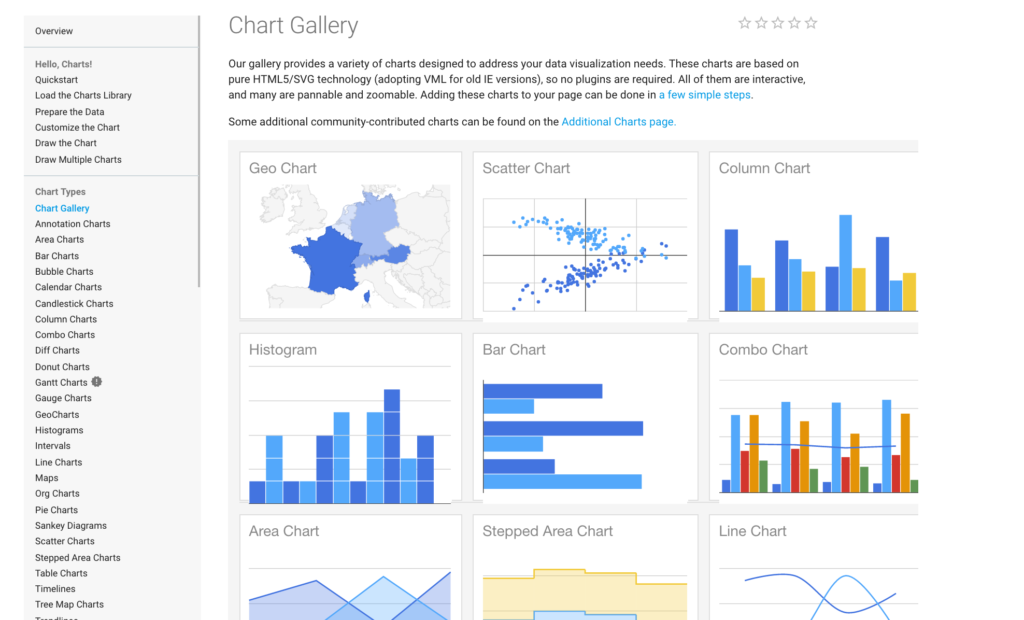
Each chart needs the following code in the head of the document to connect to the Google Charts API. We’ll start with a simple example. See simple.html in the Glitch project.
Notice that this code is included in the head section.
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script>
In addition, the code will need to know what charting package to use. Pie, bar, column and line charts are all part of the “core” package. Maps are part of the “map” package. This is included where needed in the code sample.
google.charts.load('current', {'packages':['corechart']});
We also need to use the following code to indicate what happens when the page loads, which function should be called on load. Typically, a version of the chart is drawn when page loads, so that something is visible. However, if you don’t want anything visible on load, this line can be eliminated. This is included where needed in the code sample.
google.charts.setOnLoadCallback(drawChart);
Within the function, you use JavaScript to get the values from the form into your variables, either from direct input or via JSON. The arrayToDataTable includes the elements and data (which we will replace with variable names in our example). The code ends with the declarations to create and draw the charts to the appropriate DOM element.
var chart = new google.visualization.PieChart(document.getElementById('mychart'));
chart.draw(data, options);
Chart with JSON Data
Take a look at the chart.html page on our example site (PhDigital Exercises Project on Glitch). Click on the Chart link on the site and have that open in the browser so we can refresh when we make changes. Google Charts can be used with JavaScript to pass values into charts based on user input. Instead of using values in the code, you will create a series of variables. This example shows how to get a user’s input from a form and have it create a Line Chart. When the user submits the form, the inputs are converted to variables and the values associated with those variables populate the chart.
The style section of the page includes the following code. This identifies the height of the chart. It can be adjusted to your liking. And you can use any other styles to control your page, but we’ll just go with this for now.
<style>
#yourchart {
height: 400px;
}
</style>
Included in the html section is the following code. This creates a form named “form1”, creates an onchange event to call the function to draw the chart (will be defined below) and creates a dropdown with an id of “thedropdown” to get the user’s selection. It creates a div with the id “yourchart” where the chart will be drawn.
<form id="form1" onchange="drawChart()" >
<select id="thedropdown">
<option value="cases">Cases</option>
<option value="deaths">Deaths</option>
</select>
</form>
<div id="yourchart"></div>
You will notice a script section below the HTML. This is where we will write the JavaScript to control the charts. References to the corechart pack and to the Callback (what function should be called onLoad) are already included. We will create the drawChart function below.
<script>
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
//rest of script will go here
</script>
Include the script below the html in the file within the <script> section (place it above the resize function, we’ll discuss below). This will define the chart and read data from a JSON section into it. JSON stands for JavaScript Object Notation and is a convenient format for storing data using key-value pairs. Comments in the code describe what each section is doing.
// this is the function that draws the chart
function drawChart() {
// gets the value of the dropdown
mymeas = document.getElementById("thedropdown").value;
//convert first letter to upper case
mymeas_upper = mymeas.charAt(0).toUpperCase() + mymeas.slice(1);
//json data by state for cases and deaths each month
info = [
{ "state": "Texas", "measure": "cases", "Jan": 602433, "Feb": 277323, "Mar": 139747, "Apr": 99222, "May": 58681, "Jun": 46031 },
{ "state": "Texas", "measure": "deaths", "Jan": 9087, "Feb": 6707, "Mar": 4588, "Apr": 1866, "May": 1270, "Jun": 845 },
];
//for loop reads through the data, finds a match with the dropdown value and populates the variables with the data for that value.
for(i=0; i<info.length; i++) {
if(mymeas == info[i].measure) {
Jan_tx = info[i].Jan;
Feb_tx = info[i].Feb;
Mar_tx = info[i].Mar;
Apr_tx = info[i].Apr;
May_tx = info[i].May;
Jun_tx = info[i].Jun;
}// end meas if
} // end for
//reads the data into the chart
var data = google.visualization.arrayToDataTable([
['Month', "Texas"],
['Jan', Jan_tx ],
['Feb', Feb_tx],
['Mar', Mar_tx],
['Apr', Apr_tx],
['May', May_tx],
['Jun', Jun_tx],
]);
var options = {
title: '2021 Covid ' + mymeas_upper + ' by Month',
};
//creates the new chart and identifies which element to place it
var chart = new google.visualization.LineChart(document.getElementById('yourchart'));
// draws the chart with the data and options provided.
chart.draw(data, options);
} // end function
Change Chart Type
You can change the chart type in the visualization statement. We are using timed data, so a line chart is probably best, but if you want, change the world “LineChart” to “ColumnChart” in the visualization statement. Pie, Bar, Line and Column Charts are in the same core package, so all you have to change is the chart type. How does your chart change? If you wanted to include more states on different lines or columns, you add state data to your json and add columns to the data table. You will need to adjust your script to assign the values to the variables for each state that it encounters in the json. This is just an example to show you what is possible, but once you understand the logic of JavaScript, the world of interactivity opens up to you.
For some charts, you may want to remove the legend and set min and max values for this Column Chart (keep in mind that this sets the min and max for all versions of the chart, so consider the values). You do this in the options section. For example,
legend: 'none',
vAxis: { viewWindow: { min: 0, max: 700000 }},
The Google Chart API provides numerous options that you can find in their documentation. Full detail on how to customize the code for each chart type is found on the Google Chart Gallery. These Google Charts are responsive when you use the window.onresize function. Size the window to test the resize functionality.
// this function makes the chart responsive; include the name of the proper drawChart function; if more than one chart on page, include additional statements for each drawChart function
window.onresize = function() {
drawChart();
}
Now your application has a chart.html page. You can add content throughout the site to make this a complete news application with quiz and chart! Navigate through your project, make sure everything works as intended and troubleshoot any issues. You can add additional charts to the page, but you must make sure you provide unique names for additional functions, additional dropdowns and ids for where the chart is to be drawn.
Submit Your Site: When you are done with both JavaScript exercises, you can submit the url to your site to the #webdevelopment channel.
Note: Of course, in order for others on the web to see your projects, your files must be hosted on a web server. Glitch is a good site for practicing, but it is not meant for a permanent site (and it sometimes has performance issues). Generally, you would contract with a web host (Reclaim Hosting is a good host for students, but there are many web hosts from which to choose), get access to a domain and use an ftp program (file transfer protocol) to place a copy of your files on the server. Then they are available for the world to view. Using folders and subdomains allows you to organize your work and host multiple projects on a single domain.
Final Example – Scraping a website using your knowledge of HTML/CSS. This is just a demo, but you can see how the world of coding has opened up for you!
Coding Resources
- HTML & CSS: Design and Build Websites book by Jon Duckett
- JavaScript and JQuery: Interactive Front-End Web Development book by Jon Duckett
- Mozilla Developer Network HTML Reference
- Mozilla Developer Network CSS Reference
- CodeActually.com – this is my own site where I host code samples and projects
- Codecademy – great learn-to-code resource.
- If your university provides access to LinkedIn Learning, this is also a good resource that provides access to thousands of video lessons and tutorials. It does provide a free month and after that you can renew on a monthly or annual basis.
- Glitch – Glitch is a useful resource and active community for anyone coding. It is an environment of projects that provides code samples. You can host projects on it. You can also set up and share projects so that students can code together in class. Learn more about Glitch on this post What Is Glitch?
- Reclaim Hosting – we use this company for student domains and site hosting. They have good customer support and decent pricing. There are many other web hosting options, so depending on what you want students to get from their sites (custom domain, support for other platforms like WordPress, etc), you can determine which hosting service is best.
- Stack Overflow – I find the answers to most of my coding problems on Stack Overflow. However, I usually land there from a Google search of my problem.
- GitHub – GitHub is like a social network for code. Developers share projects and code on this site.
- Google Charts Exercise – CodeActually.com – additional exercises using the Google Charts API can be found on this link, including Maps and GeoCharts, with additional exercises and suggestions for including Multiple Charts on a page.
- Filtering Data Exercise – CodeActually.com exercise that demonstrates how to loop over json data and present in a table.
Module Evaluation
After each module, you will be asked to do an evaluation. Go to the link for the PhDigital 2022 Zoom Modules Evaluation and select the module you are reviewing. This is the July 1 Module. Please do this as soon as you can after the Zoom session.