Google Charts Exercise
The Google Charts API provides code that you can use, with your knowledge of JavaScript, to make interactive charts. Look through the extensive Google Chart Gallery for ideas about charts you’d might be able to use. We’ll be working with the pie and column charts.
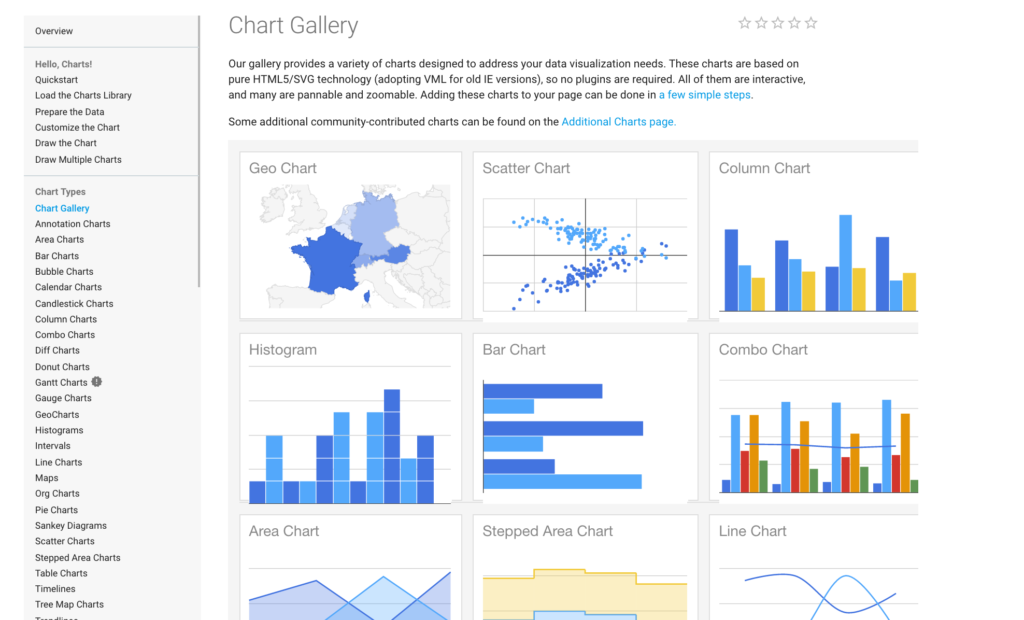
Each chart needs the following code in the head of the document to connect to the Google Charts API. Let’s put this code in a file named chart.html. Use the index.html page as a template and Save As, chart.html. Include the code below in the head section.
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script>
In addition, the code will need to know what charting package to use. Pie, bar, column an and line charts are all part of the “core” package. Maps are part of the “map” package. This is included where needed in the code samples below.
google.charts.load('current', {'packages':['corechart']});
We also need to use the following code to indicate what happens when the page loads, which function should be called on load. Typically, a version of the chart is drawn when page loads, so that something is visible. However, if you don’t want anything visible on load, this line can be eliminated. This is included where needed in the code samples below.
google.charts.setOnLoadCallback(drawChart);
Then you create the function that draws the chart. In these examples, the function is named drawChart(), but you can name it anything you want as long as that is how you call it. You call it in the form HTML with an appropriate event. For example:
<form id="form1" onsubmit="drawChart(); return false" >
Within the function, you use JavaScript to get the values from the form into your variables, either from direct input or via JSON. The arrayToDataTable includes the elements and variable names. The code ends with the declarations to create and draw the charts to the appropriate DOM element.
var chart = new google.visualization.PieChart(document.getElementById('piechart'));chart.draw(data, options);
The examples below provide working code for various types of charts.
Pie Chart with JSON Data
Google Charts can be used with JavaScript to pass values into charts based on user input. Instead of using values in the code, you will create a series of variables. This example shows how to get a user’s input from a form and have it create a Pie Chart. When the user submits the form, the inputs are converted to variables and the values associated with those variables populate the chart.
Start by including the following items to style your chart. Include the code in the style section. This identifies the height of the chart. It can be adjusted to your liking.
<style>
#yourchart {
height: 400px;
}
</style>
Include the following in the html section in the <div class=”col”>. This creates a form named “form1”, creates an onchange event to all the function to draw the chart (will be defined below) and creates a dropdown with an id of “thedropdown” to get the user’s selection. It creates a div with the id “yourchart” where the chart will be drawn.
<form id="form1" name="form1" onchange="drawChart()" >
<select id="thedropdown">
<option value="male">Male</option>
<option value="female">Female</option>
</select>
</form>
<div id="yourchart"></div>
Include the script below the html in the file. This will define the chart and read data from a JSON section into it. JSON stands for JavaScript Object Notation and is a convenient format for storing data using key-value pairs. Comments in the code describe what each section is doing.
<script>
//these are required to establish the chart package and determine which function to call when the page loads
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
// this is the json area that includes the data that will be read into the chart
info = [{gender: "male", work: 40, eat: 8, commute: 4, tv: 3, sleep: 40},
{gender: "female", work: 45, eat: 9, commute: 3, tv: 5, sleep: 50}
];
// this is the function that draws the chart
function drawChart() {
// gets the value of the dropdown
mygender = document.getElementById("thedropdown").value;
//for loop reads through the data, finds a match with the dropdown value and populates the variables with the data for that value.
for(i=0; i<info.length; i++) {
if(mygender == info[i].gender) {
work = info[i].work;
eat = info[i].eat;
commute = info[i].commute;
tv = info[i].tv;
sleep = info[i].sleep;
}// end if
} // end for
//reads the data into the chart
var data = google.visualization.arrayToDataTable([
['Task', 'Hours per Week'],
['Work', work],
['Eat', eat],
['Commute', commute],
['Watch TV', tv],
['Sleep', sleep]
]);
var options = {
title: 'Weekly Activities',
colors: ['orange','#00cc33', 'pink', 'yellow','lightblue'],
};
//creates the new chart and identifies which element to place it
var chart = new google.visualization.PieChart(document.getElementById('yourchart'));
// draws the chart with the data and options provided.
chart.draw(data, options);
}
</script>
Change to Column Chart
Change the type of chart in the visualization statement to a ColumnChart. Pie, Bar, Line and Column Charts are in the same core package, so all you have to change is the chart type. How does your chart change? You may want to remove the legend and set min and max values for this Column Chart. Make sure each line is separated by a comma. Full detail on how to customize the code for each chart type is found on the Google Chart Gallery.
legend: 'none',
vAxis: { viewWindow: { min: 0, max: 50 }},
These Google Charts are responsive. Size the window and refresh the browser. They don’t size along with the browser, but on a smaller device, they will come up appropriately in the window.
Now your application has a chart.html page. You can add content throughout the site to make this a complete news application with quiz and chart! Navigate through your project, make sure everything works as intended and troubleshoot any issues.
Try It with New Data
Let’s try one with some different data. Dockless Mobility has become all the rage in Austin. They have also created a lot of problems, with scooters and bikes being left all over the sidewalks and people not using them correctly. The city of Austin provides information about Dockless Mobility 311 calls on their data portal. I created a dataset by searching for “Dockless Mobility” and then generating a csv that I opened in Excel. I removed all columns except date and zip code. I generated the actual month and created a pivot table that summarized the data by zip and month for the top five zip codes. Then created a csv that could be used in a csv-to-json converter to create the JSON we needed for the chart. Use this data to make a column chart that uses a dropdown to change the zipcode and shows each month on the chart. Modify the code you used above. Be sure to change the vAxis to accommodate this new data.
info = [ { "zip": 78701, "Jan": 64, "Feb": 110, "Mar": 354, "Apr": 325, "May": 268 }, { "zip": 78704, "Jan": 98, "Feb": 100, "Mar": 252, "Apr": 126, "May": 76 }, { "zip": 78703, "Jan": 35, "Feb": 42, "Mar": 119, "Apr": 82, "May": 27 }, { "zip": 78705, "Jan": 24, "Feb": 36, "Mar": 71, "Apr": 80, "May": 16 }, { "zip": 78702, "Jan": 21, "Feb": 30, "Mar": 60, "Apr": 30, "May": 24 } ]
Additional Resources
Google Charts Exercise – CodeActually.com – additional exercises using the Google Charts API can be found on this link, including Maps and GeoCharts, with additional exercises and suggestions for including Multiple Charts on a page.
Filtering Data Exercise – CodeActually.com exercise that demonstrates how to loop over json data and present in a table.